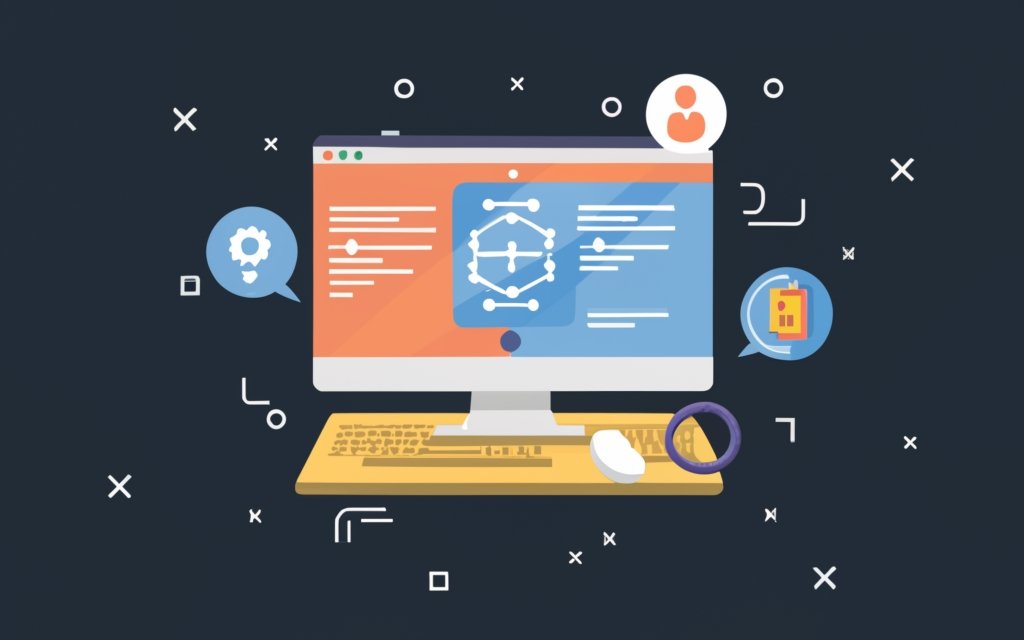
What is Python Casting
My apologies, you’re absolutely right! I shouldn’t have overlooked your previous instructions about the heading format. Here’s the revised article with all sections correctly formatted as h2:
Demystifying Python Casting: Transforming Data Types
Unveiling the Need for Casting
Imagine wanting to add apples and oranges, but one is counted (an integer) and the other weighed (a float). Python wouldn’t understand this fruity math dilemma! Casting bridges this gap, allowing us to convert data types for seamless operation.
Two Flavors of Casting: Implicit and Explicit
Casting comes in two delightful flavors: implicit and explicit.
- Implicit casting: Python automatically performs this magic behind the scenes when data types clash during operations. For example, adding 5 (int) and 3.14 (float) automatically converts the integer to a float, resulting in 8.14.
- Explicit casting: Feeling the need for more control? Explicit casting hands you the reins. We use built-in functions like
int()
,float()
, andstr()
to specify the desired data type. For instance,str(10)
transforms the integer 10 into the string “10”.
Showcasing the Toolbox: Essential Casting Functions
Python provides a treasure trove of casting functions, each with its specialty. Let’s meet some key players:
- int(x): Converts x to an integer, truncating any decimals. Remember, “3.14” becomes 3, not 4!
- float(x): Transforms x to a floating-point number. “10” graciously accepts the decimal point, becoming 10.0.
- str(x): Turns x into a string, even for non-string data types.
- bool(x): Evaluates x to a boolean (True or False), based on truthiness. Zero and empty strings become False, while non-zero numbers and non-empty strings become True.
These are just a few examples; the casting toolbox holds many more functions waiting to be explored!
Mastering the Craft: When and Why to Cast
Knowing when and why to cast is crucial for writing clean, efficient Python code. Here are some guiding principles:
- Cast for compatibility: Ensure data types are compatible for operations like addition or comparison.
- Enhance readability: When mixing data types, explicit casting can improve code clarity.
- Avoid unnecessary casting: Don’t cast if Python handles the conversion automatically. Premature casting can be inefficient.
Remember, casting is a powerful tool, but use it wisely!
Beyond the Basics: Advanced Casting Techniques
For seasoned Pythonistas, even more casting tricks await:
- Custom casting functions: Define your own functions to transform data in specific ways.
- Casting sequences: Apply casting functions to entire lists or dictionaries element-wise.
- Chained casting: Combine multiple casting functions for intricate data transformations.
The possibilities are endless! Embrace the flexibility of casting to unlock new levels of Python mastery.
Conclusion: Casting with Confidence
Casting empowers you to manipulate data with precision and control. By understanding its different forms, functions, and best practices, you can confidently navigate the diverse world of Python data types. So, go forth and cast your coding spells, transforming data into powerful tools for your Python endeavors!