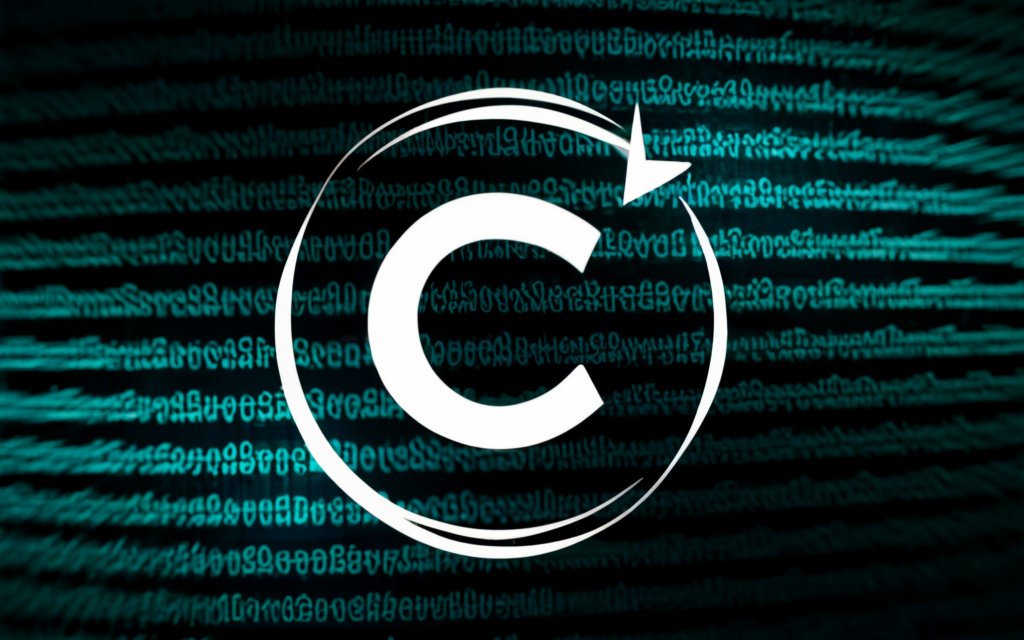
C Program to Print Alternate Prime Numbers from 1 to n
Sifting through numbers to find prime gems can be a tedious task, but with the right tool, it becomes an elegant exercise in C programming. This article delves into the world of printing alternate prime numbers from 1 to n in C, exploring efficient algorithms, practical code examples, and optimization techniques to help you master this numerical challenge.
1. Understanding the Challenge: Beyond Consecutive Primes
Finding all prime numbers within a range is a common programming task, but printing only every other one adds an intriguing twist. This requires not only identifying individual primes but also keeping track of their occurrence and printing them accordingly.
2. Algorithm Strategies: Sieving for Efficiency
Two primary algorithms tackle this challenge efficiently:
- Naive Approach: Loop through all numbers from 1 to n, check for primality using individual checks (divisibility by smaller primes), and print every other prime encountered. This method, while simple, can be computationally expensive for large values of n.
C
for (int i = 1; i <= n; i++) {
int is_prime = 1; // Assume prime
for (int j = 2; j <= i / 2; j++) { // Check divisibility by smaller primes
if (i % j == 0) {
is_prime = 0; // Not prime
break;
}
}
if (is_prime && (i % 2 != 0 || i == 2)) { // Print every other prime
printf("%d ", i);
}
}
- Sieve of Eratosthenes: This optimized algorithm creates a boolean array to mark composite numbers (non-prime) based on multiples of known primes. By iteratively eliminating multiples, the remaining unmarked numbers are guaranteed to be prime. This approach significantly reduces the number of primality checks needed, making it more efficient for larger ranges.
C
#define MAX_SIZE 10000 // Adjust based on desired range
int is_prime[MAX_SIZE];
void sieve(int n) {
memset(is_prime, 1, sizeof(is_prime)); // Mark all as prime initially
for (int i = 2; i <= sqrt(n); i++) { // Loop for known primes
if (is_prime[i]) {
for (int j = i * i; j <= n; j += i) { // Mark multiples as composite
is_prime[j] = 0;
}
}
}
}
int main() {
int n;
printf("Enter upper limit (n): ");
scanf("%d", &n);
sieve(n);
int count = 0;
for (int i = 2; i <= n; i++) {
if (is_prime[i] && count % 2 == 0) { // Print every other prime
printf("%d ", i);
count++;
}
}
printf("\n");
return 0;
}
Both algorithms achieve the desired result, but the Sieve of Eratosthenes offers significant performance gains for larger datasets.
3. Optimization Techniques: Fine-tuning Your Code
Beyond choosing the right algorithm, additional optimizations can further improve your program’s efficiency:
- Limit Sieve Size: Instead of creating a sieve for the entire range, calculate the maximum prime needed based on the upper limit (n) and use a smaller sieve size.
- Precompute Primes: Store a list of known prime numbers beforehand to avoid repeated primality checks during the sieving process.
- Utilize Bit Manipulation: Use bit operations to mark and access elements in the sieve array for improved space and time efficiency.
These techniques, coupled with careful algorithm selection, can significantly boost your program’s performance, especially when dealing with large numbers.
4. Beyond the Basics: Exploring Variations
The core concept of printing alternate primes can be extended to explore further challenges:
- Printing nth Alternate Prime: Modify the code to print the nth alternate prime number instead of all of them.
- Finding Primes within a Specific Range: Adapt the program to find alternate primes within a specific sub-range within the overall limit (n).
- Combining with Other Number Theory Concepts: Integrate this algorithm with other number theory concepts like finding twin primes or prime factorization to create more complex and engaging programs.
These variations encourage experimentation and application of your newfound understanding of prime number identification and manipulation.
5. Conclusion: Mastering the Art of Sieving
Printing alternate prime numbers in C might seem like a simple task at first, but by understanding the underlying algorithms and optimization techniques, you can write efficient and elegant code that can handle even the most challenging datasets.
This article provided a comprehensive overview of the topic, covering the basic concepts, algorithms, and optimization techniques. By practicing with the provided code examples and exploring the suggested variations, you can master the art of sieving and become a master of prime number identification.
Here are some additional tips to help you master this challenging task:
- Practice makes perfect. The more you practice, the better you will become at identifying and implementing efficient algorithms.
- Don’t be afraid to experiment. Try different approaches and see what works best for you.
- Use online resources. There are many helpful resources available online, including websites, forums, and tutorials.
With practice and dedication, you can master the art of sieving and become a master of prime number identification. So what are you waiting for? Start practicing today!