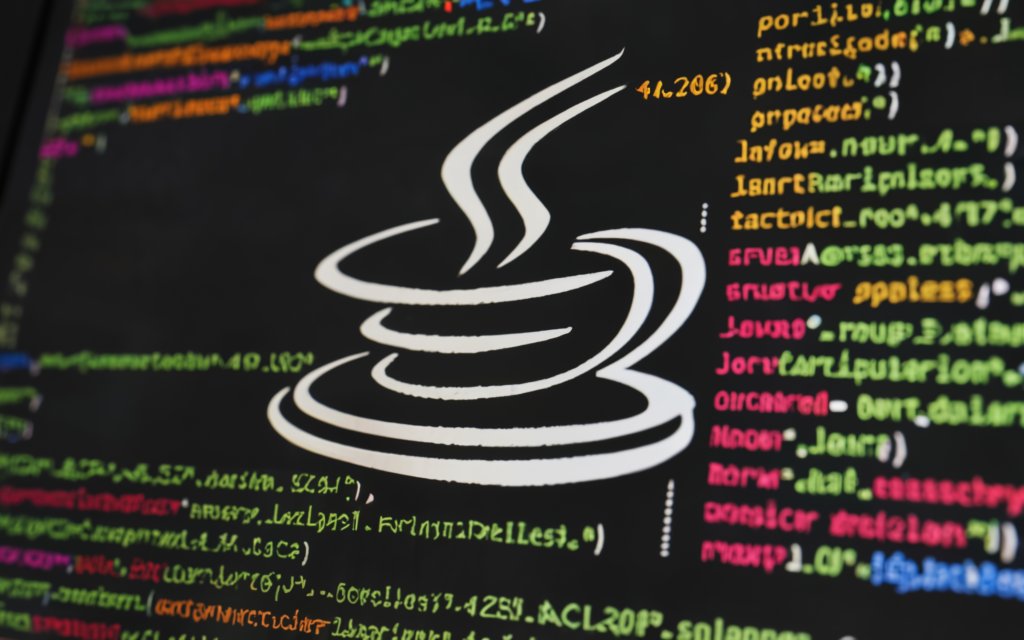
Generic Class Example in Java
Generic classes in Java unleash a new level of flexibility and power when working with different data types. They transcend the limitations of single-type classes by allowing you to specify a placeholder, or type parameter, that can be filled with various types later. This article delves into the world of generic classes in Java, exploring their core concepts, practical examples, and advanced techniques to equip you with this invaluable tool for robust and versatile code.
1. Demystifying Generics: Unveiling the Type Parameter
At the heart of a generic class lies the type parameter. Think of it as an empty box; you can later fill it with any appropriate data type, like Integer, String, or even your own custom class. This provides tremendous flexibility, allowing you to write code that can work with different types without the need for multiple, redundant versions.
Java
public class GenericBox<T> {
private T value;
public void setValue(T value) {
this.value = value;
}
public T getValue() {
return value;
}
}
In this example, GenericBox<T>
defines a generic class with a type parameter T
. This essentially creates a class template that can be instantiated with any type later. You can use T
within the class methods, allowing you to store, retrieve, and manipulate objects of the specified type.
2. Putting it to Practice: Instantiating Generic Classes
The true power of generics shines when you instantiate the generic class with specific types. Remember the empty box? Now you can fill it!
Java
GenericBox<String> stringBox = new GenericBox<>(); // Box for Strings
stringBox.setValue("Hello World!"); // Store a String
String message = stringBox.getValue(); // Retrieve the String
GenericBox<Integer> intBox = new GenericBox<>(); // Box for Integers
intBox.setValue(123); // Store an Integer
int number = intBox.getValue(); // Retrieve the Integer
Here, you create two boxes: one for Strings and another for Integers. The same GenericBox class seamlessly adapts to accommodate both types, showcasing its versatility.
3. Beyond the Basics: Advanced Techniques
The world of generics extends beyond simple type parameters. Explore these advanced techniques to unlock their full potential:
- Multiple Type Parameters: Define multiple type parameters for even more flexibility (e.g.,
Pair<K, V>
holds a key-value pair). - Type Bounds: Specify restrictions on the type parameter (e.g.,
Box<T extends Number>
requires T to be a subclass of Number). - Generic Methods: Apply generics to methods within a class for even more generalized functionality.
- Wildcards: Use wildcard characters (
?
) to represent unknown types or relax type restrictions for specific scenarios.
These techniques enhance the power and flexibility of generic classes, allowing you to create truly adaptable and robust code.
4. Benefits and Applications: Why Go Generic?
Using generics offers numerous benefits:
- Improved Code Reusability: Write code that works with various types without redundancy, making it cleaner and more maintainable.
- Enhanced Type Safety: The compiler checks for type compatibility at compile time, preventing runtime errors and ensuring data integrity.
- Increased Flexibility and Adaptability: Adapt your code to different needs and data types seamlessly without rewriting significant portions.
Generic classes find applications in diverse areas:
- Collections: Java’s core collections like ArrayList and HashMap utilize generics to store and manage various data types efficiently.
- APIs and Libraries: Many libraries and frameworks leverage generics for flexibility and ease of use.
- Custom Data Structures: You can create your own generic data structures to handle diverse data needs in your projects.
5. Conclusion: Unlocking the Power of Generics in Java
Mastering generic classes empowers you to write elegant, flexible, and adaptable code. By embracing this powerful tool, you can elevate your programming skills, tackle complex challenges with ease, and create robust solutions that can seamlessly adapt to changing needs.
Remember, the journey with generics is a continuous learning experience. Explore advanced techniques, practice with diverse applications, and stay updated with the latest advancements in the Java ecosystem. As you delve deeper into this world, you’ll unlock a universe of possibilities, where flexible and versatile code becomes your signature, and data types simply become the raw materials you sculpt into efficient and elegant solutions.
So, embrace the power of generics, code with confidence, and unlock the full potential of your Java programming skills! Remember, the journey of mastering generics is a continuous adventure, filled with exciting possibilities and endless creations. Keep coding, keep learning, and let your code become a testament to the adaptability and elegance that defines a true Java master.