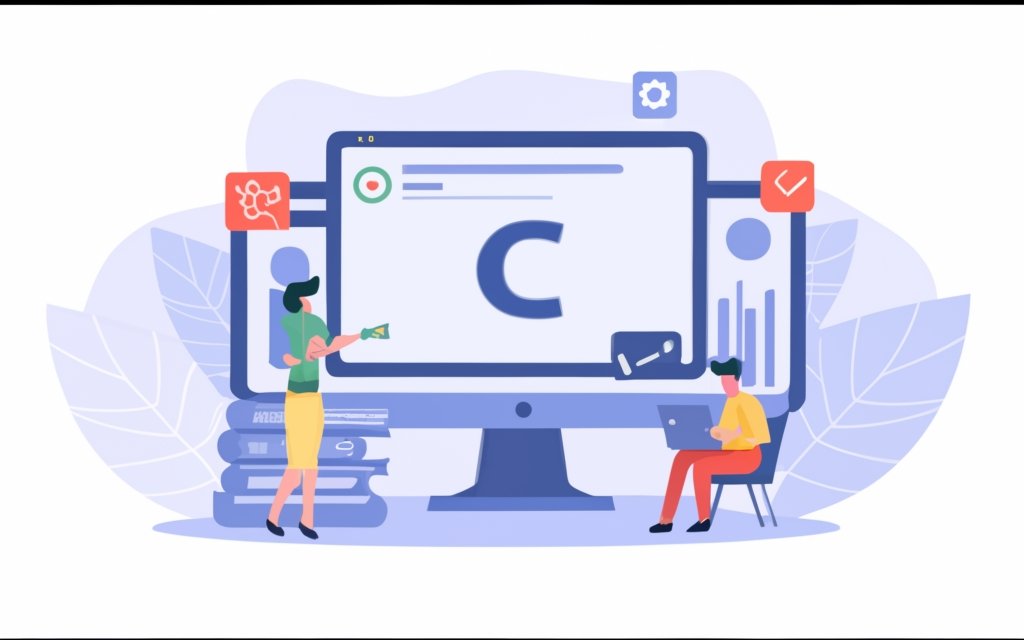
C (programming language)
Series Programs in C Exercises and Solutions
Introduction
- Series programs in C involve generating sequences of numbers based on specific patterns or rules.
- They serve as essential practice for:
- Understanding loops
- Implementing conditional logic
- Using mathematical formulas
- Enhancing problem-solving skills
Common Series Exercises
Here are several common series exercises along with their solutions:
1. Arithmetic Series
- Generate an arithmetic series where each term is obtained by adding a constant difference (common difference) to the previous term.
C
#include <stdio.h>
int main() {
int a = 2, d = 3, n = 5; // First term, common difference, number of terms
printf("Arithmetic Series: ");
for (int i = 1; i <= n; ++i) {
printf("%d ", a);
a += d; // Calculate the next term
}
printf("\n");
return 0;
}
2. Geometric Series
- Generate a geometric series where each term is obtained by multiplying the previous term by a constant ratio.
C
#include <stdio.h>
int main() {
int a = 2, r = 3, n = 5; // First term, common ratio, number of terms
printf("Geometric Series: ");
for (int i = 1; i <= n; ++i) {
printf("%d ", a);
a *= r; // Calculate the next term
}
printf("\n");
return 0;
}
3. Fibonacci Series
- Generate the Fibonacci series where each term is the sum of the two preceding terms, starting from 0 and 1.
C
#include <stdio.h>
int main() {
int n = 10; // Number of terms
int t1 = 0, t2 = 1, nextTerm;
printf("Fibonacci Series: ");
for (int i = 1; i <= n; ++i) {
printf("%d ", t1);
nextTerm = t1 + t2;
t1 = t2;
t2 = nextTerm;
}
printf("\n");
return 0;
}
4. Prime Number Series
- Print prime numbers within a given range.
C
#include <stdio.h>
int main() {
int lower = 1, upper = 50;
printf("Prime Numbers between %d and %d:\n", lower, upper);
for (int num = lower; num <= upper; ++num) {
int isPrime = 1;
for (int i = 2; i <= num / 2; ++i) {
if (num % i == 0) {
isPrime = 0;
break;
}
}
if (isPrime) {
printf("%d ", num);
}
}
printf("\n");
return 0;
}
Additional Exercises
- Explore other series exercises such as:
- Factorial series
- Palindrome series
- Square series
- Cube series
- Even/odd series
- Incorporate user input for flexibility.
- Experiment with different loop structures and techniques.
- Challenge yourself with more complex series patterns.