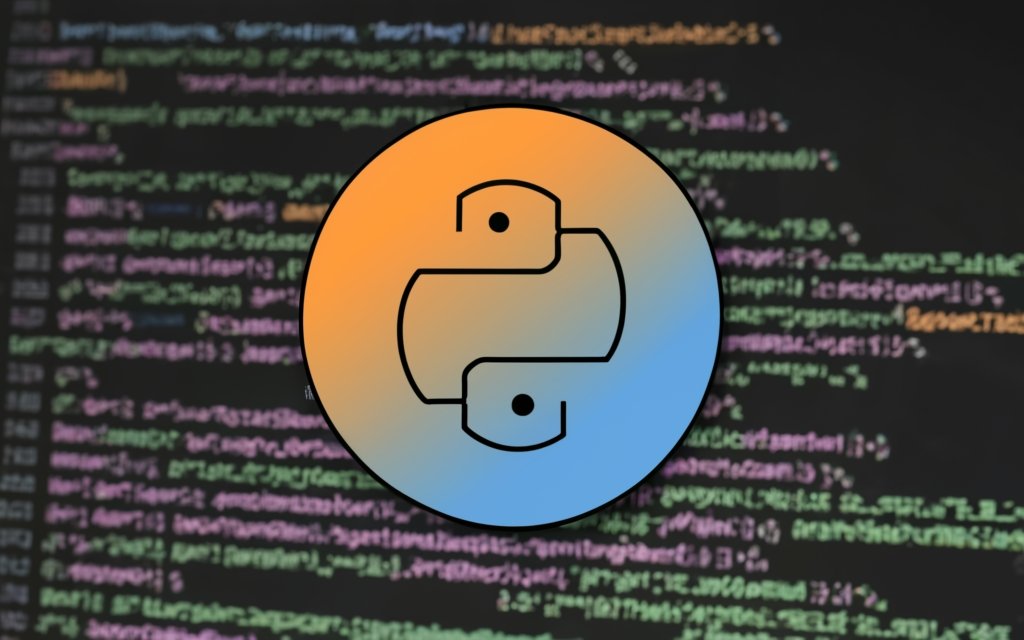
Understanding Different Types of Numbers in Python
Python, like many other programming languages, deals with various kinds of numbers. Each type has its own characteristics and is suited for different situations. This guide delves into the three primary numeric data types in Python: integers, floats, and complex numbers.
Integers: Whole Numbers Without Fractions
Integers represent whole numbers, positive or negative, without any decimal or fractional parts. They are typically used for counting, indexing, and other operations where precise, non-divisible values are needed.
- Examples: 10, -5, 0, 123456789
- Operations: Integers support all basic arithmetic operations (+, -, *, //, %), comparisons (<, >, <=, >=, ==, !=), and logical operations (and, or, not).
- Code Example:
Python
a = 10
b = 5
c = a + b
print(f"Sum of {a} and {b} is: {c}")
x = 7
y = 2
z = x // y # Integer division discards the remainder
print(f"Integer division of {x} by {y} is: {z}")
- Output:
Sum of 10 and 5 is: 15
Integer division of 7 by 2 is: 3
Floats: Numbers with Decimal Places
Floats, or floating-point numbers, represent real numbers with decimal parts. They are used for calculations involving fractions, measurements, and other situations where precise fractional values are necessary.
- Examples: 3.14, -1.2345, 10.0, 0.5
- Operations: Floats support all basic arithmetic operations, comparisons, and logical operations similar to integers. However, be aware of rounding errors inherent in their representation.
- Code Example:
Python
pi = 3.14
radius = 5
area = pi * radius**2
print(f"Area of a circle with radius {radius} is: {area}")
temperature = 37.5
is_fever = temperature > 37.0
print(f"Is {temperature} considered a fever? {is_fever}")
- Output:
Area of a circle with radius 5 is: 78.5
Is 37.5 considered a fever? True
Complex Numbers: Beyond the Real
Complex numbers extend the realm of numbers beyond real numbers to include imaginary numbers. Imaginary numbers involve the unit j
, representing the square root of -1. Complex numbers are used in advanced scientific and engineering applications.
- Examples: 1+2j, 3-5j, (2+3j) + (1-j)
- Operations: Complex numbers support addition, subtraction, multiplication, and division with specific rules for handling real and imaginary components. Built-in functions like
abs()
andcmath
module provide advanced functionalities. - Code Example:
Python
c1 = 1 + 2j
c2 = 3 - 5j
c3 = c1 + c2
print(f"Sum of complex numbers {c1} and {c2} is: {c3}")
modulus = abs(c1)
print(f"Modulus (absolute value) of {c1} is: {modulus}")
- Output:
Sum of complex numbers (1+2j) and (3-5j) is: (4-3j)
Modulus (absolute value) of (1+2j) is: 2.23606797749979
These are just the fundamental types of numbers in Python. Beyond these, there are specialized data types like fractions
and libraries like numpy
that provide extended functionalities for numerical computations. Remember to choose the appropriate type based on your specific needs and desired precision.
This guide serves as a starting point for your exploration of the diverse world of numbers in Python. Feel free to delve deeper into each type and experiment with them in your code!