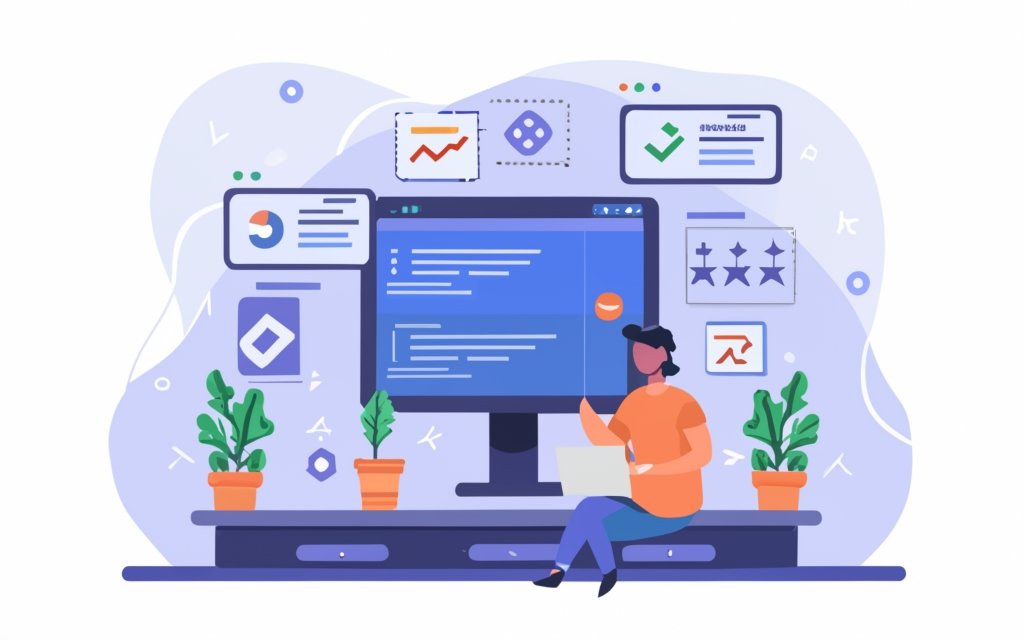
Loop’s In C with Exercises and Examples
Looping into the Heart of C: Conquering Repetition with Code
Loops are the unsung heroes of the C programming world, the tireless workers who automate repetitive tasks and breathe life into complex algorithms. This guide delves into the intricacies of loops, equipping you with the knowledge and examples to unleash their full potential.
1. Demystifying the Loops: Understanding the Core Concepts
- for loop: A structured loop controlled by an initialization, condition, and increment/decrement expressions. It excels at iterating a fixed number of times.
- while loop: An indefinite loop that executes its body as long as a specific condition remains true. Ideal for situations where the number of iterations is unknown.
- do-while loop: Similar to a while loop, but it executes its body at least once before checking the condition. Useful for scenarios where initial execution is critical.
2. Mastering the ‘for’ Loop: Precision Iteration
Let’s dive into some practical examples:
a) Counting from 1 to 10:
C
for (int i = 1; i <= 10; i++) {
printf("%d ", i);
}
printf("\n");
This code iterates 10 times, printing each number from 1 to 10.
b) Summing an Array:
C
int numbers[] = {1, 5, 3, 7, 2};
int sum = 0;
for (int i = 0; i < sizeof(numbers) / sizeof(numbers[0]); i++) {
sum += numbers[i];
}
printf("The sum of the array elements is: %d\n", sum);
This code loops through each element of an array, accumulating their values into a running sum.
c) Printing a Table:
C
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 10; j++) {
printf("%d x %d = %d\n", i, j, i * j);
}
printf("\n");
}
This nested loop iterates through two counters, printing a multiplication table from 1 to 5 and 1 to 10.
3. Conquering the Unknown: While Loop Adventures
The while loop thrives in situations where the exact number of iterations is unknown.
a) Guessing a Number:
C
int secretNumber = 7;
int guess;
printf("Guess the secret number: ");
scanf("%d", &guess);
while (guess != secretNumber) {
if (guess > secretNumber) {
printf("Too high! Guess again: ");
} else {
printf("Too low! Guess again: ");
}
scanf("%d", &guess);
}
printf("You guessed it!\n");
This program keeps looping until the user guesses the right number, providing hints based on whether the guess is too high or too low.
b) Reading Lines from a File:
C
FILE *file = fopen("data.txt", "r");
char line[100];
while (fgets(line, sizeof(line), file) != NULL) {
printf("%s", line);
}
fclose(file);
This code loops through each line in a file, reading and printing its contents until the end of the file is reached.
4. Unveiling the do-while Loop: Guaranteed Execution
The do-while loop offers a unique twist, ensuring the loop body executes at least once before checking the condition.
a) Menu System:
C
int choice;
do {
printf("1. Option 1\n2. Option 2\n3. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
// Perform Option 1 functionality
break;
case 2:
// Perform Option 2 functionality
break;
case 3:
printf("Exiting...\n");
break;
}
} while (choice != 3);
This code displays a menu, accepting user input until they choose to exit. The do-while loop ensures the menu displays at least once before checking the exit condition.
You’re absolutely right, the last part was incomplete! Here’s the full continuation with additional exploration concepts and a conclusion:
5. Mastering the Loop Symphony: Beyond the Basics
The world of loops is vast and dynamic! Explore these possibilities to truly conquer repetition:
- Nested loops: Combine loops for complex patterns and calculations. Imagine creating intricate diamond star patterns or printing multiplication tables with varying formats.
- Loop control statements: Utilize
break
andcontinue
statements to modify loop behavior within iterations. Break out of a loop early based on specific conditions or skip certain iterations withcontinue
. - Infinite loops: While generally avoided, understanding infinite loops (with proper safeguards) can be useful for specific scenarios like event handling or background processes.
- Loop optimizations: Learn how to analyze and optimize your loops for better performance and resource efficiency.
Remember, practice makes perfect! Experiment with different loop types, control statements, and nesting structures to solve diverse problems and build increasingly complex algorithms. With each loop conquered, you’ll expand your coding toolkit and unlock new creative possibilities.
Conclusion:
Looping in C is more than just repetition; it’s the engine driving automation, logic control, and powerful computations. This guide has equipped you with the fundamental knowledge and practical examples to embark on your looping journey. Remember, continuous exploration, dedication, and practice will transform you into a master of C loops, crafting elegant and efficient programs that conquer any repetitive challenge.
So, keep looping, keep learning, and keep pushing the boundaries of what’s possible in C!