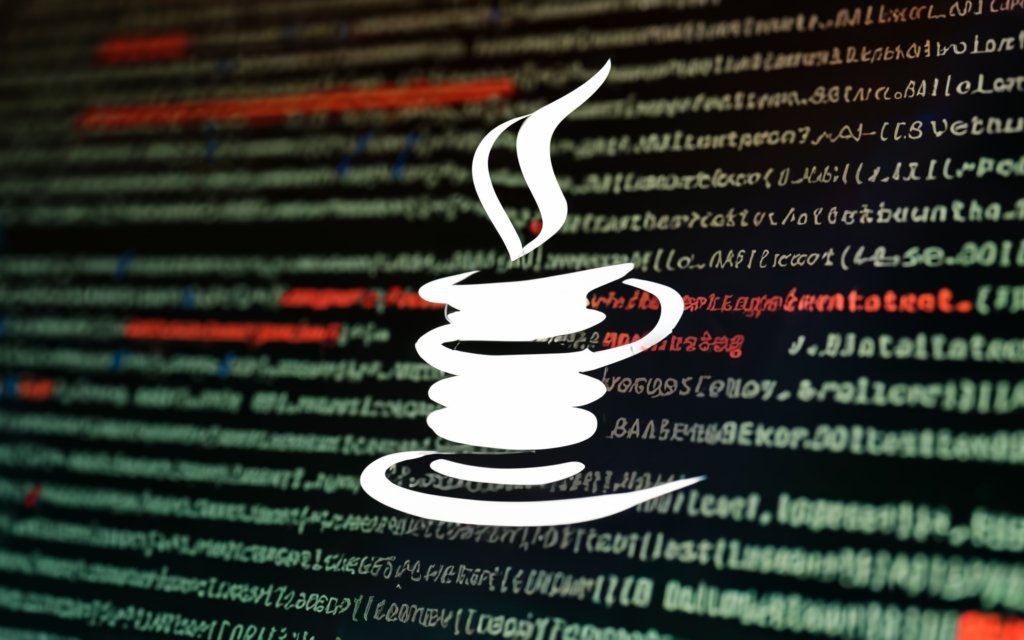
Java (programming language)
Java Program to Print Square of Given Array Elements
Introduction
This guide will explore the process of creating a Java program that effectively calculates and prints the squares of elements within a given array. We’ll delve into the code structure, logic, and key steps involved in implementing this task.
Key Concepts
- Arrays in Java: Arrays are fundamental data structures that store a fixed-size sequential collection of elements of the same data type.
- Looping: Iteration over array elements is crucial for accessing and manipulating individual values.
- Mathematical Operations: The square of a number is calculated by multiplying it by itself.
Steps to Implement
- Import Necessary Class:
Java
import java.util.Arrays; // For array manipulation
- Declare Array and Variables:
Java
int[] numbers = {1, 2, 3, 4, 5}; // Example array
int[] squares = new int[numbers.length]; // Array to store squares
- Calculate Squares Using a Loop:
Java
for (int i = 0; i < numbers.length; i++) {
squares[i] = numbers[i] * numbers[i]; // Calculate square and store
}
- Print the Squares:
Java
System.out.println("Original Array: " + Arrays.toString(numbers));
System.out.println("Squares of Array Elements: " + Arrays.toString(squares));
Complete Example Code:
Java
import java.util.Arrays;
public class SquareArrayElements {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
int[] squares = new int[numbers.length];
for (int i = 0; i < numbers.length; i++) {
squares[i] = numbers[i] * numbers[i];
}
System.out.println("Original Array: " + Arrays.toString(numbers));
System.out.println("Squares of Array Elements: " + Arrays.toString(squares));
}
}
Explanation
- The
import java.util.Arrays;
statement enables the use of theArrays
class for array-related operations. - Two arrays are declared:
numbers
to store the original values andsquares
to store the calculated squares. - The
for
loop iterates through each element in thenumbers
array. - Inside the loop, the square of the current element is calculated and stored in the corresponding index of the
squares
array. - The
Arrays.toString()
method is used to print the arrays in a readable format.
Key Points to Remember
- The loop iterates through the array using the
i
variable as an index to access elements. - The
squares[i] = numbers[i] * numbers[i];
statement performs the squaring operation and stores the result. - Ensure that the
squares
array has the same length as thenumbers
array to avoid index-out-of-bounds errors.