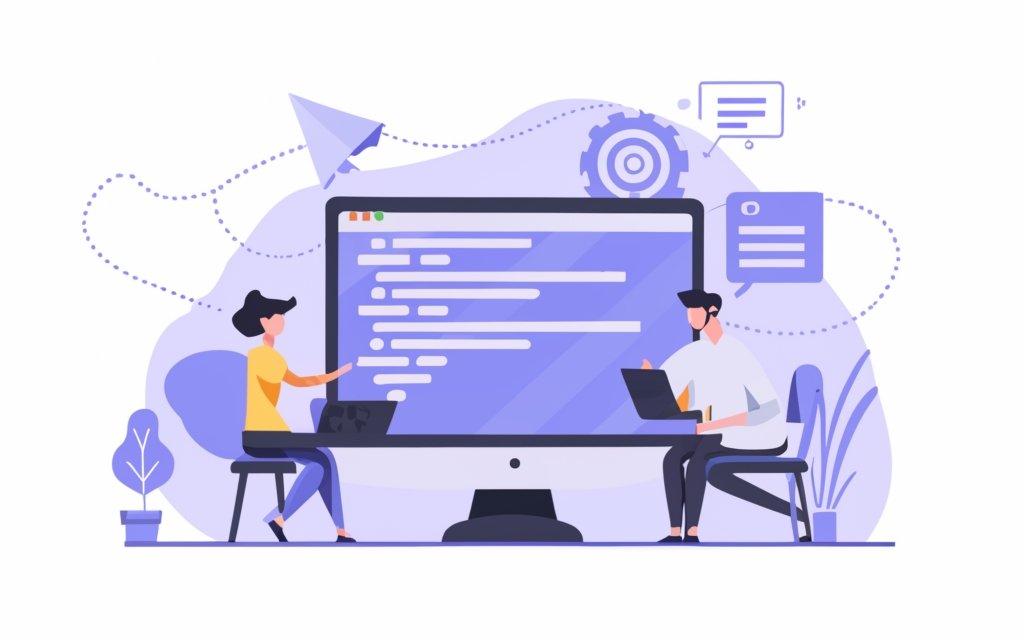
C (programming language)
C star pattern programming exercises and examples
Introduction
- Star patterns in C programming involve arranging asterisks (
*
) in various shapes and patterns using loops and conditional statements. - They serve as excellent practice for beginners to:
- Grasp loop constructs
- Enhance logical thinking
- Develop problem-solving skills
- Practice basic C syntax
Common Star Patterns
Here are examples of common star patterns, along with their C code implementations:
1. Right-angled Triangle
C
#include <stdio.h>
int main() {
int rows = 5;
for (int i = 1; i <= rows; ++i) {
for (int j = 1; j <= i; ++j) {
printf("*");
}
printf("\n");
}
return 0;
}
2. Inverted Right-angled Triangle
C
#include <stdio.h>
int main() {
int rows = 5;
for (int i = rows; i >= 1; --i) {
for (int j = 1; j <= i; ++j) {
printf("*");
}
printf("\n");
}
return 0;
}
3. Pyramid
C
#include <stdio.h>
int main() {
int rows = 5;
for (int i = 1; i <= rows; ++i) {
for (int j = 1; j <= rows - i; ++j) {
printf(" ");
}
for (int j = 1; j <= 2 * i - 1; ++j) {
printf("*");
}
printf("\n");
}
return 0;
}
4. Inverted Pyramid
C
#include <stdio.h>
int main() {
int rows = 5;
for (int i = rows; i >= 1; --i) {
for (int j = 1; j <= rows - i; ++j) {
printf(" ");
}
for (int j = 1; j <= 2 * i - 1; ++j) {
printf("*");
}
printf("\n");
}
return 0;
}
5. Diamond
C
#include <stdio.h>
int main() {
int rows = 5;
for (int i = 1; i <= rows; ++i) {
for (int j = 1; j <= rows - i; ++j) {
printf(" ");
}
for (int j = 1; j <= 2 * i - 1; ++j) {
printf("*");
}
printf("\n");
}
for (int i = rows - 1; i >= 1; --i) {
for (int j = 1; j <= rows - i; ++j) {
printf(" ");
}
for (int j = 1; j <= 2 * i - 1; ++j) {
printf("*");
}
printf("\n");
}
return 0;
}
Further Exploration
- Experiment with different patterns: hollow shapes, numbers, letters, etc.
- Explore nested loops for intricate patterns.
- Incorporate user input for row and column numbers.
- Challenge yourself with complex patterns.
- Use visual aids for better understanding (if available).