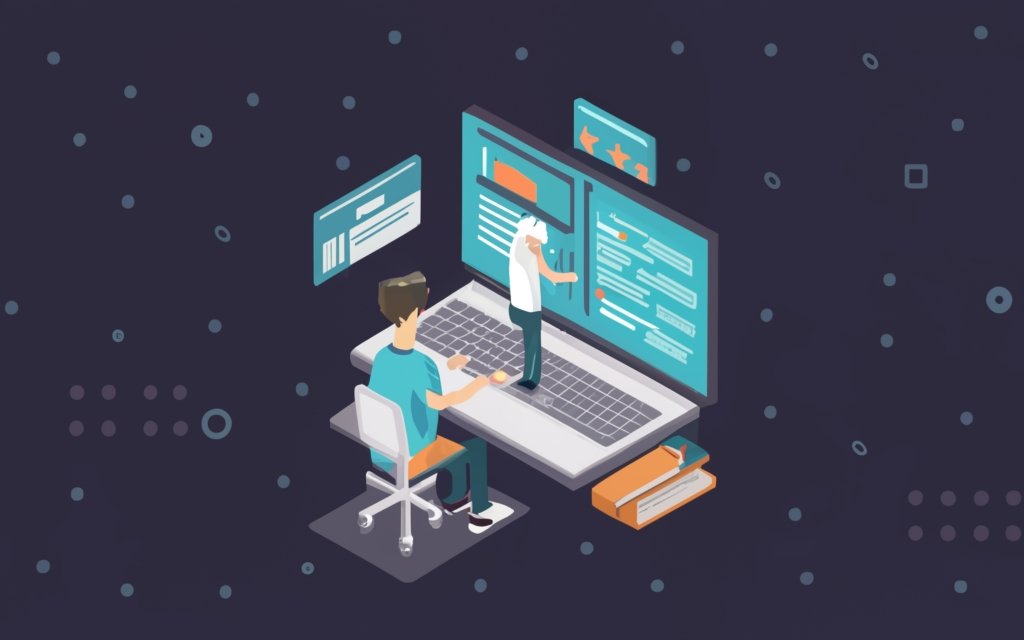
C (programming language)
C program using do-while loop to check inside or outside
Understanding the Problem
- Goal: Write a C program that takes coordinates (x, y) from the user and determines whether the point lies inside or outside a circle of radius 5 centered at (0, 0).
- Key Concepts:
- Loops: do-while loop for repeated input and checking.
- Conditional statements: if-else for decision-making.
- Mathematical calculations: Distance formula for checking distance from the center.
- Input/Output (I/O): scanf for input, printf for output.
Step-by-Step Guide
1. Header Inclusion
C
#include <stdio.h>
#include <math.h> // For sqrt() function
- Explanation: Include the standard input/output library and math library.
2. Main Function
C
int main() {
// Code statements here
return 0;
}
3. do-while Loop for Repeated Input
C
do {
// Get coordinates from the user
int x, y;
printf("Enter coordinates (x, y): ");
scanf("%d %d", &x, &y);
// Calculate distance from the center
double distance = sqrt(pow(x, 2) + pow(y, 2)); // Distance formula
// Check if inside or outside
if (distance <= 5) {
printf("Point (%d, %d) is inside the circle.\n", x, y);
} else {
printf("Point (%d, %d) is outside the circle.\n", x, y);
}
// Ask if the user wants to continue
char choice;
printf("Do you want to check another point? (y/n): ");
scanf(" %c", &choice); // Note the space before %c to consume newline
} while (choice == 'y' || choice == 'Y');
4. Explanation of Code:
- do-while loop: Ensures at least one iteration of input and checking.
- scanf(“%d %d”, &x, &y): Reads the coordinates entered by the user.
- distance = sqrt(pow(x, 2) + pow(y, 2)): Calculates the distance from the point to the center using the distance formula.
- if-else statement: Determines whether the distance is less than or equal to 5 (inside) or greater than 5 (outside).
- printf statements: Print the result to the console.
- scanf(” %c”, &choice): Reads the user’s choice to continue or not (note the space before %c to consume the newline character).
5. Complete Code
C
#include <stdio.h>
#include <math.h>
int main() {
do {
int x, y;
printf("Enter coordinates (x, y): ");
scanf("%d %d", &x, &y);
double distance = sqrt(pow(x, 2) + pow(y, 2));
if (distance <= 5) {
printf("Point (%d, %d) is inside the circle.\n", x, y);
} else {
printf("Point (%d, %d) is outside the circle.\n", x, y);
}
char choice;
printf("Do you want to check another point? (y/n): ");
scanf(" %c", &choice);
} while (choice == 'y' || choice == 'Y');
return 0;
}
6. Executing the Program
- Save the code as a
.c
file (e.g.,inside_outside.c
). - Compile using a C compiler (e.g.,
gcc inside_outside.c -o inside_outside
). - Run the executable (e.g.,
./inside_outside
).
I hope this guide is helpful!