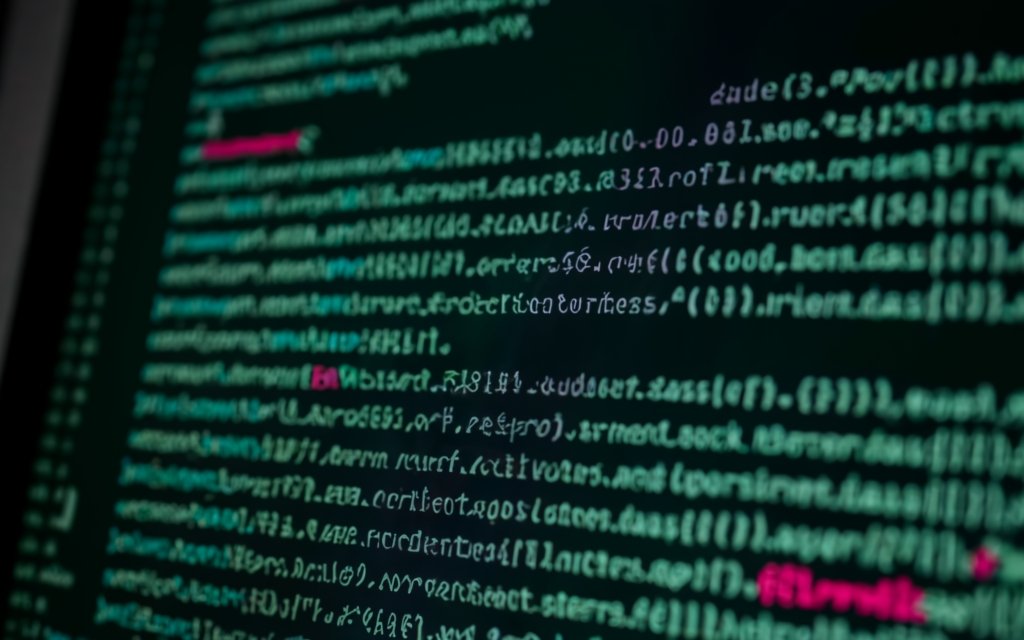
C (programming language)
C Program to Show How to Handle Pointers
Understanding Pointers in C
- Pointers: Variables that store memory addresses, providing direct access to data stored at those addresses.
- Key Concepts:
- Declaration: Using the
*
operator to declare pointer variables. - Dereferencing: Accessing the value at a memory address using the
*
operator. - Address-of operator:
&
to get the memory address of a variable. - Pointer arithmetic: Performing operations on pointers to navigate memory.
- Declaration: Using the
Step-by-Step Guide
1. Header Inclusion
C
#include <stdio.h>
- Explanation: Includes the standard input/output library for functions like
printf
.
2. Main Function
C
int main() {
// Code statements here
return 0;
}
3. Pointer Declaration
C
int num = 10; // Regular integer variable
int *ptr; // Pointer variable to hold an integer address
- Explanation:
num
: Declares an integer variable with value 10.ptr
: Declares a pointer variable that can hold the address of an integer.
4. Assigning Address to Pointer
C
ptr = # // Assigning the address of num to ptr
- Explanation: The
&
operator gets the memory address ofnum
and assigns it toptr
.
5. Accessing Value Through Pointer (Dereferencing)
C
printf("Value of num: %d\n", *ptr); // Accessing the value at the address pointed to by ptr
- Explanation: The
*
operator dereferencesptr
, accessing the value stored at the memory address it points to.
6. Modifying Value Through Pointer
C
*ptr = 20; // Modifying the value at the address pointed to by ptr
printf("New value of num: %d\n", num); // Changes will reflect in the original variable
- Explanation: Modifying the value using
*ptr
directly changes the value ofnum
in memory.
7. Pointer Arithmetic
C
int arr[] = {1, 2, 3, 4, 5}; // Array declaration
int *arrPtr = arr; // Pointer pointing to the first element of the array
printf("Second element: %d\n", *(arrPtr + 1)); // Accessing elements using pointer arithmetic
printf("Address of third element: %p\n", arrPtr + 2); // Adding offsets to pointers
- Explanation: Pointer arithmetic allows navigating through arrays using pointers.
8. Complete Code Example
C
#include <stdio.h>
int main() {
int num = 10;
int *ptr = #
printf("Value of num: %d\n", *ptr);
*ptr = 20;
printf("New value of num: %d\n", num);
int arr[] = {1, 2, 3, 4, 5};
int *arrPtr = arr;
printf("Second element: %d\n", *(arrPtr + 1));
printf("Address of third element: %p\n", arrPtr + 2);
return 0;
}
9. Essential Pointer Concepts
- Null Pointers: Pointers that don’t point to any valid memory address, often used to indicate the end of data structures or errors.
- Pointers and Functions: Passing pointers to functions allows functions to modify variables in the calling scope.
- Dynamic Memory Allocation: Using pointers to allocate and deallocate memory dynamically during program execution (e.g., with
malloc
andfree
).
Remember that pointers are powerful but can also lead to errors if not used carefully. Practice and understanding are key to mastering pointer manipulation in C.