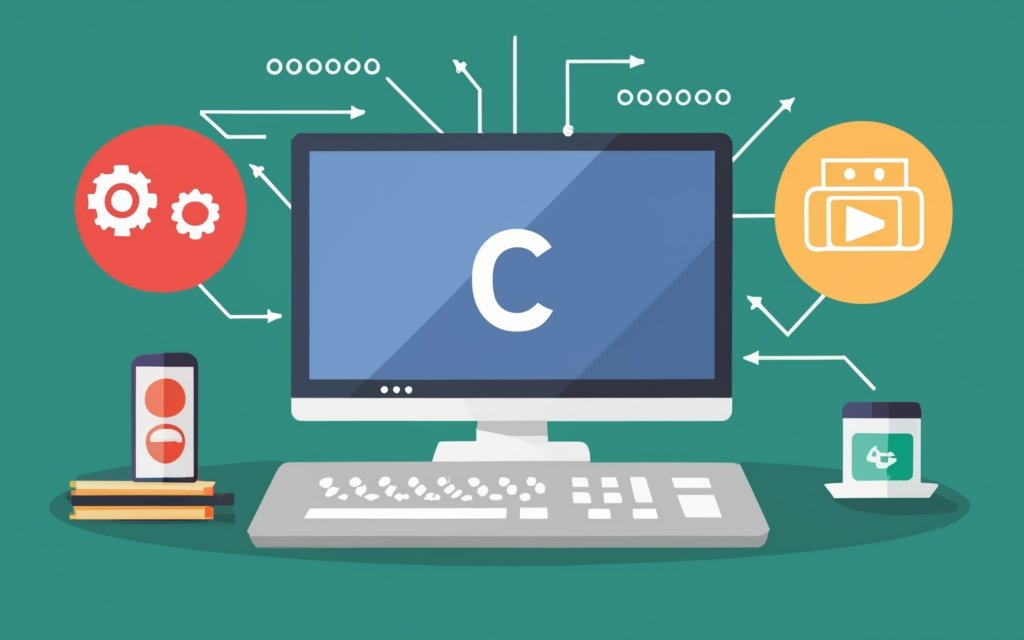
C program to separate the individual characters from a string
Deciphering the Code: Separating Characters in a C String
Ever wondered how to dissect a string in C, extracting its individual characters like building blocks? This guide sheds light on various methods for achieving this seemingly simple yet versatile task, equipping you with the knowledge and code to break down strings with confidence.
1. Looping Through the Array: A Classic Approach
C strings are essentially character arrays terminated by a null character (\0
). Understanding this fundamental structure unlocks the first method: iterating through the array to access each character.
C
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
int i;
// Loop through each character until the null terminator
for (i = 0; str[i] != '\0'; i++) {
printf("%c ", str[i]); // Print each character
}
printf("\n");
return 0;
}
This code loop iterates from index 0 (first character) until it encounters the null terminator, accessing and printing each character within the string.
2. Pointer Power: Navigating the String
Another approach leverages pointers to traverse the string memory. By manipulating and dereferencing a pointer, you can efficiently access and print each character.
C
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
char *ptr = str; // Initialize pointer to start of string
// Loop while the pointer points to a valid character
while (*ptr != '\0') {
printf("%c ", *ptr); // Print the character pointed to
ptr++; // Increment the pointer to next character
}
printf("\n");
return 0;
}
This code uses a pointer variable (ptr
) initialized to the beginning of the string (str
). The loop iterates as long as the character pointed to (accessed with *ptr
) is not the null terminator. Each iteration prints the character and increments the pointer to move to the next element in the string.
3. Built-in Function Finesse: Utilizing strchr
For a concise solution, C offers the strchr
function, which locates the first occurrence of a specified character (including \0
) within a string.
C
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char *ptr;
// Find the first occurrence of null terminator
ptr = strchr(str, '\0');
// Loop backwards, printing characters until string beginning
while (ptr != str) {
printf("%c ", *ptr);
ptr--;
}
printf("\n");
return 0;
}
This code leverages strchr
to find the null terminator. Starting from there, it uses a loop that decrements the pointer and prints each character until it reaches the beginning of the string (str
).
4. Beyond the Basics: Exploring Variations and Applications
The ability to isolate and manipulate individual characters opens a doorway to exciting possibilities:
- Reverse a string: Utilize any of the methods and iterate through the string in reverse order, printing each character.
- Count specific characters: Modify the loop conditions to count occurrences of a specific character (e.g., vowels) within the string.
- Extract substrings: Leverage pointer manipulation or string functions to extract specific sections of the string based on desired indices or delimiters.
Remember, the best approach depends on your specific needs and coding style. Experiment with different methods and refine your skills to tackle diverse string manipulation challenges with confidence.
Conclusion: Decoding the String Universe
Separating characters in C is not just about individual components; it’s about understanding the internal structure of strings and unlocking their potential for creative manipulation and data analysis. This guide has equipped you with fundamental methods and highlighted further exploration avenues. Embrace the possibilities, delve deeper into the string universe, and let your C code sing with clarity and precision!
I hope this in-depth guide provides comprehensive knowledge and inspires you to explore the diverse applications of character separation in your C programming endeavors!