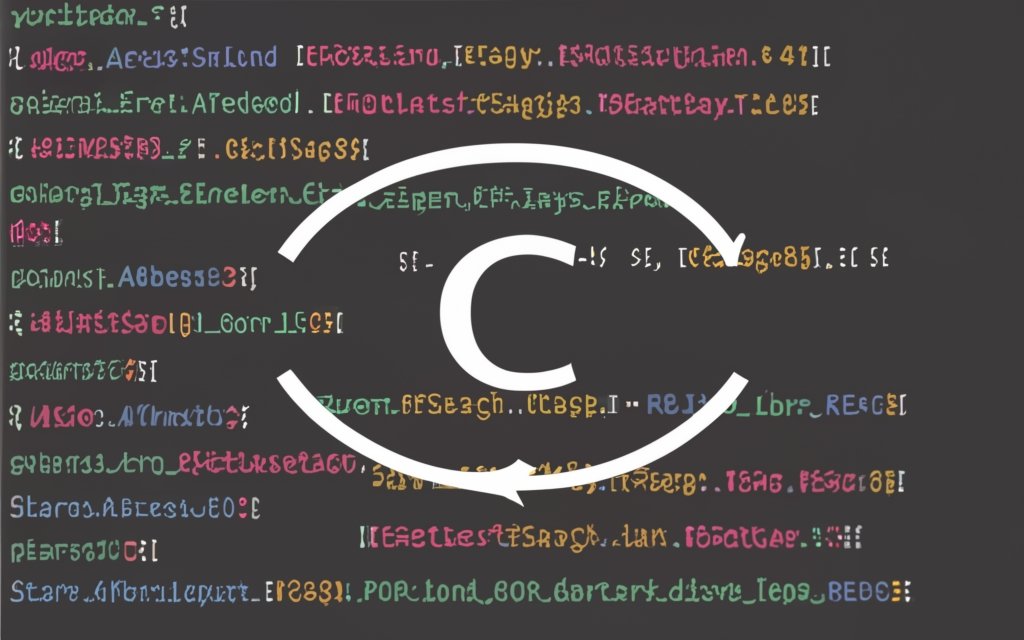
C Program To Print Maximum Number Between Two Numbers without using if statement
Finding the Champion: Printing the Maximum Number without “if” in C
The humble “if” statement, while a cornerstone of conditional logic, isn’t the only way to determine the bigger value. This guide explores a C program that finds the maximum between two numbers using alternative approaches, showcasing the flexibility and creativity of coding.
1. Setting the Stage: Variables and Operators
C
int num1, num2, max;
We declare three integer variables:
num1
andnum2
: To store the user-defined numbers.max
: To hold the final maximum value.
Let’s also introduce some helpful operators:
- Ternary Operator (?:): Acts as a condensed “if-else” statement, evaluating conditions and assigning based on the outcome.
- Bitwise Operators: These operate on individual bits of the binary representation, offering unique approaches for comparison.
2. User Input: Gathering the Numbers
C
printf("Enter the first number: ");
scanf("%d", &num1);
printf("Enter the second number: ");
scanf("%d", &num2);
We prompt the user for two numbers, storing them in num1
and num2
for further processing.
3. Option 1: Using the Ternary Operator
C
max = (num1 > num2) ? num1 : num2;
This single line leverages the power of the ternary operator. It acts like a condensed “if-else” statement:
- If
num1 > num2
(condition is true),max
is assignednum1
. - Otherwise,
max
is assignednum2
.
The result is concise and elegant, demonstrating a powerful alternative to traditional branching statements.
4. Option 2: Utilizing Bitwise XOR and AND
C
max = (num1 ^ num2) & (num1 - num2 >> 31);
This approach uses two key operators:
- Bitwise XOR (^): This operator performs logical “exclusive OR” on each bit of its operands. In this case,
num1 ^ num2
returns 1 only for the bit where one operand is 1 and the other is 0. - Bitwise AND (&): This operator performs logical “AND” on each bit of its operands. Here,
(num1 ^ num2) & (num1 - num2 >> 31)
becomes:1 & 0
whennum1 > num2
, resulting in 0 (meaningnum1
wins).1 & 1
whennum1 < num2
, resulting in 1 (meaningnum2
wins).
This method might appear cryptic at first, but it utilizes bit-level manipulation to achieve the same result as the ternary operator and avoids branching.
5. Displaying the Champion
C
printf("The maximum number is: %d\n", max);
Finally, we use printf
to announce the grand champion – the number stored in max
.
6. Beyond the Basics: Exploring Other Paths
- Min/Max Functions: The C standard library offers
min()
andmax()
functions that directly achieve this comparison. - Advanced Bitwise Techniques: Explore further bitwise operations for performing comparisons and calculations in unique ways.
Remember, choosing the best approach depends on your specific needs and preferences. While “if” statements remain a valuable tool, understanding alternative techniques enhances your programming vocabulary and opens doors to creative solutions. So, keep exploring and push the boundaries of what’s possible in C!