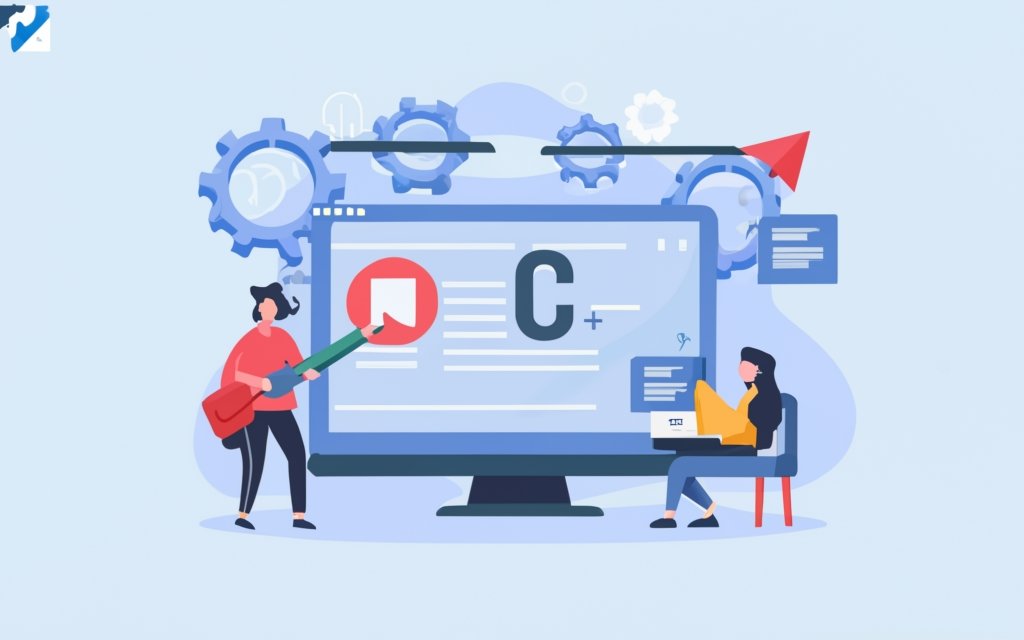
C Program to print hollow diamond star pattern
Shimmering Diamonds: Crafting a Hollow Star Pattern in C
There’s something captivating about a hollow diamond pattern. Its glistening outline of stars against a background of darkness evokes a sense of elegance and intrigue. This guide delves into crafting a C program that brings this visual delight to life, enabling you to print captivating hollow diamond star patterns of any desired size.
Building Blocks: Loops and Logic
Our journey begins with two trusty companions:
- Loops: We’ll leverage nested loops to meticulously control the placement of stars and spaces, shaping the diamond’s form.
- Conditional Statements: These act as guiding lights, dictating when to print a star and when to leave a space, ensuring the hollow center and defined edges.
Step 1: Setting the Stage
C
#include <stdio.h>
int size; // Stores the user-defined pattern size
We include the stdio.h
library for user interaction and declare an integer variable, size
, to hold the desired size of the diamond pattern.
Step 2: User Input: Defining the Diamond’s Dimensions
C
printf("Enter the size of the hollow diamond pattern: ");
scanf("%d", &size);
Prompt the user to enter the desired size of the diamond, accepting the input as an integer and storing it in size
.
Step 3: Outer Loop – Shaping the Diamond’s Upper Half
C
for (int i = 1; i <= size; i++) { // Loop iterates 'size' times, building rows from top to center
// ...
}
This loop lays the foundation for the diamond’s upper half. It iterates size
times, controlling the vertical construction of rows from top to the centermost line.
Step 4: Inner Loop – Star Placement and Hollow Center
C
for (int j = 1; j <= 2 * size - 1; j++) { // Loop iterates for each column within each row
if (i == 1 || i == size || (j <= size - i + 1) && (j >= size + i - 1)) { // Conditional for printing stars
printf("*");
} else { // Conditional for printing spaces
printf(" ");
}
}
Nested within the outer loop lies the inner loop, responsible for star placement within each row. It iterates for each column (2 * size – 1) to cover the entire diamond width.
- The conditional statement determines when to print a star:
- If it’s the first (top) or last (bottom) row, or
- If the current column falls within the diagonal boundaries defined by
size - i + 1
andsize + i - 1
(accounting for the hollow center).
- Otherwise, a space is printed to maintain the hollow diamond shape.
Step 5: Line Break and Loop Completion
C
printf("\n"); // Move to new line after completing each row
}
After iterating through all columns in a row, the printf("\n")
statement adds a line break, moving to the next row for star placement.
The outer loop continues until all rows (specified by size
) are completed, forming the upper half of the diamond.
Step 6: Reflecting the Diamond: Lower Half Construction
C
for (int i = size - 1; i >= 1; i--) { // Loop iterates 'size' times, building rows from center to bottom
// ...
}
To complete the diamond, we mirror the process for the lower half. This loop iterates size
times in reverse order, building rows from the centermost line down to the base.
C
for (int j = 1; j <= 2 * size - 1; j++) { // Loop iterates for each column within each row
// ...
}
The inner loop remains the same, handling star placement and spaces based on the conditional statements and reflecting the diamond’s symmetry.
Step 7: Witnessing the Starry Gem
Compile and run the program! Enter the desired size, and behold the majestic hollow diamond star pattern sparkle on your screen, a testament to your programming prowess.
Beyond the Basics: Adding Dazzling Touches
Once you’ve mastered the basic diamond pattern, why not elevate it to new heights? Here are some exciting ways to customize your creation:
- Variable Star Character: Swap the classic “*” with different characters like “+”, “#”, or even symbols like “@” or “&” for a unique twist on the pattern.
- Colored Stars: Embrace the rainbow! Utilize escape sequences or terminal coloring libraries like “ncurses” to inject vibrant hues into your diamond stars, painting a dazzling spectacle on your screen.
- Animated Diamonds: Infuse your diamond with life! Experiment with manipulating loop variables like increments or conditional checks to create pulsating, shimmering effects, making your diamond dance and glimmer.
- Interactive Diamonds: Take control! Allow users to input the desired size, character type, or even color palettes, transforming your program into an interactive diamond generator.
Remember: There are no limits to your creativity! Experiment, combine different techniques, and explore further options to craft your own signature diamond patterns. Who knows, your invention might become the next dazzling gem in the programmer’s toolkit!
Conclusion:
This guide has equipped you with the tools and insights to create captivating hollow diamond star patterns in C. Remember, programming is a journey of continuous learning and exploration. So, keep practicing, unleash your creativity, and watch your code blossom into dazzling visual masterpieces!
Additional Resources:
- C Primer Plus by Stephen Prata
- Head First C by David Griffiths
- Online tutorials and forums dedicated to C programming