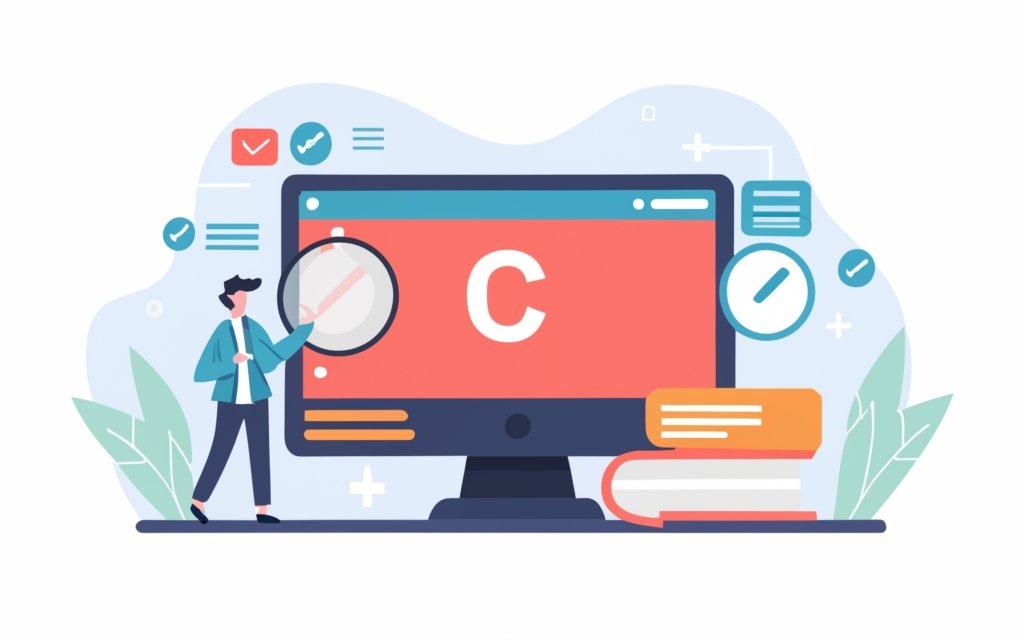
C (programming language)
C program to print half right side of pyramid star pattern
Understanding the Problem
- Goal: Write a C program that prints a half right-side pyramid pattern of stars, starting with a single star and increasing in each row to form a triangle shape.
- Key Concepts:
- Loops:
for
loops for controlled iterations. - Indentation: Aligning stars using spaces for the desired pattern.
- Output formatting:
printf
for printing stars and spaces.
- Loops:
Step-by-Step Guide
1. Header Inclusion
C
#include <stdio.h>
- Explanation: Includes the standard input/output library for functions like
printf
.
2. Main Function
C
int main() {
// Code statements here
return 0;
}
3. Get User Input
C
int rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
4. Outer Loop for Rows
C
for (int i = 1; i <= rows; i++) {
// Code for printing each row
}
5. Inner Loop for Columns
C
for (int j = 1; j <= i; j++) {
printf("*"); // Print a star
}
printf("\n"); // Move to the next line after each row
6. Indentation for Pattern
C
// Indentation for aligning stars to the right
for (int j = 1; j <= rows - i; j++) {
printf(" "); // Print two spaces for indentation
}
7. Complete Code
C
#include <stdio.h>
int main() {
int rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= rows - i; j++) {
printf(" ");
}
for (int j = 1; j <= i; j++) {
printf("*");
}
printf("\n");
}
return 0;
}
8. Executing the Program
- Save the code as a
.c
file (e.g.,half_pyramid_star.c
). - Compile using a C compiler (e.g.,
gcc half_pyramid_star.c -o half_pyramid_star
). - Run the executable (e.g.,
./half_pyramid_star
).
Example Output
Enter the number of rows: 5 * ** ***