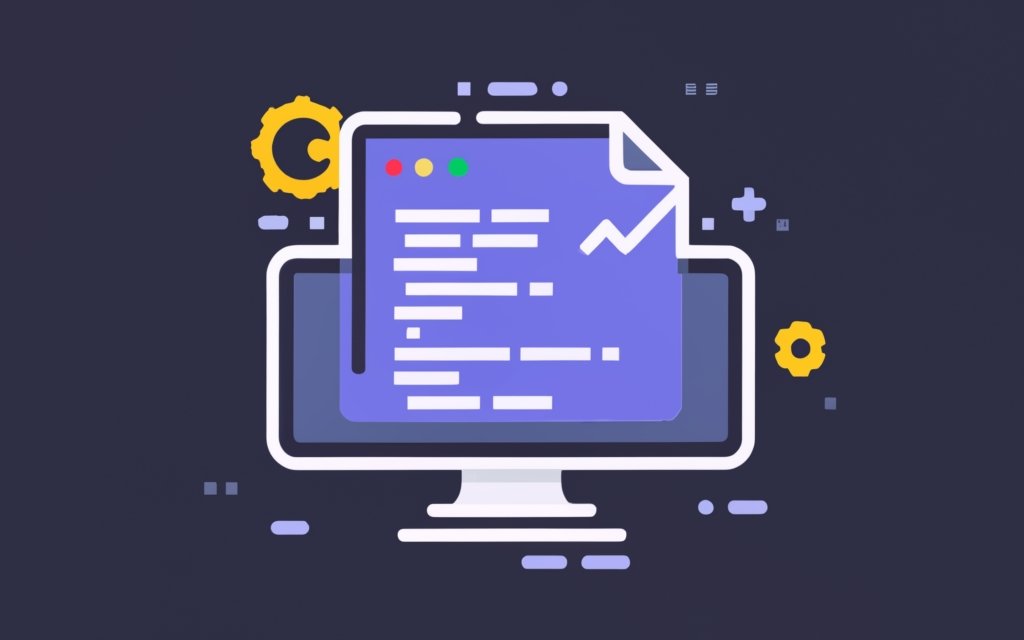
C (programming language)
C program to print half left side of the pyramid star pattern
Understanding the Pattern
- Visualize a pyramid standing upright, showcasing only its left half.
- The pyramid’s structure is formed using stars, with each row containing an increasing number of stars.
- The pattern resembles a triangle with its right side absent.
Key Concepts Involved
- Loops: Utilize loops to iterate through rows and stars, creating the pattern’s repetitive structure.
- Conditional Statements: Employ conditional statements to control the number of stars printed in each row, shaping the pyramid’s distinct form.
- Character Printing: Use the
printf
function to display the stars on the console, forming the visual pattern.
Step-by-Step Code Breakdown
- Header Inclusion:
C
#include <stdio.h>
- Main Function:
C
int main() {
- Variable Declaration:
C
int rows, i, j;
- User Input for Number of Rows:
C
printf("Enter the number of rows: ");
scanf("%d", &rows);
- Outer Loop for Rows:
C
for (i = 1; i <= rows; i++) { // Iterate from 1 to rows
- Inner Loop for Stars:
C
for (j = 1; j <= i; j++) { // Print stars based on current row number
printf("*");
}
- Newline for Next Row:
C
printf("\n");
- Return Statement:
C
return 0;
}
Full Code:
C
#include <stdio.h>
int main() {
int rows, i, j;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; i++) {
for (j = 1; j <= i; j++) {
printf("*");
}
printf("\n");
}
return 0;
}
Explanation:
- The
stdio.h
header file provides input/output functions likeprintf
andscanf
. - The
main
function is the program’s entry point. - Variables
rows
,i
, andj
are used to store the number of rows, row counter, and star counter, respectively. - The user is prompted to enter the desired number of rows.
- The outer loop iterates from 1 to the specified number of rows, creating each row of the pyramid.
- The inner loop prints stars up to the current row number, forming the left-side pyramid shape.
printf("\n")
moves the cursor to the next line, starting a new row.return 0
indicates successful program execution.
Customization and Enhancements:
- Change the printed character to create different patterns (e.g., numbers, letters, symbols).
- Allow user input for the character to be printed.
- Explore colors or blinking effects using ANSI escape sequences for visual appeal.
Conclusion:
By understanding loops, conditional statements, and character printing in C, you can create engaging visual patterns like the half left side of the pyramid star pattern. Experiment with different variations and customizations to expand your programming skills and create visually appealing outputs.