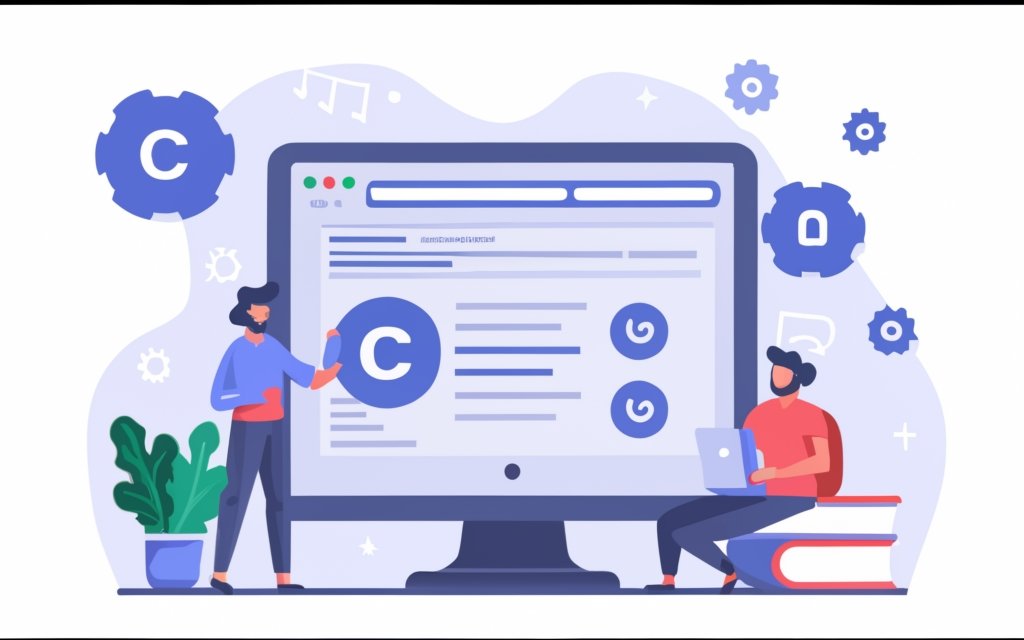
C (programming language)
C program to print 2 15 41 80 132 197 275 366 numbers
Understanding the Number Series
- This series doesn’t follow a standard arithmetic or geometric progression.
- It has a unique pattern that involves a combination of operations.
- To generate this series in C, we’ll need to determine the mathematical formula that defines it.
Deriving the Formula for the Series
- Observe the Differences:
- Calculate the differences between consecutive terms:
- 15 – 2 = 13
- 41 – 15 = 26
- 80 – 41 = 39
- 132 – 80 = 52
- Notice that the differences themselves are increasing by a constant value of 13.
- Calculate the differences between consecutive terms:
- Generalize the Formula:
- Represent the terms as
T1
,T2
,T3
, … - Based on the observed pattern, the formula can be expressed as:
T2 = T1 + 13
T3 = T2 + 26 = T1 + 13 + 26
T4 = T3 + 39 = T1 + 13 + 26 + 39
- And so on, with the difference increasing by 13 each time.
- Represent the terms as
- Simplify the Formula:
- Rewrite the formula in terms of the term number (n):
Tn = T1 + 13(n-1) + 13(n-2) + ... + 13
- Using the sum of arithmetic series formula, this simplifies to:
Tn = T1 + (13/2)(n-1)(n)
- Rewrite the formula in terms of the term number (n):
Implementation in C
C
#include <stdio.h>
int main() {
int n = 8; // Number of terms to print
printf("Number Series:\n");
for (int i = 1; i <= n; ++i) {
int term = 2 + (13/2) * (i - 1) * i; // Apply the formula
printf("%d ", term);
}
printf("\n");
return 0;
}
Explanation:
- The formula
2 + (13/2) * (i - 1) * i
is used to calculate each term. - The
for
loop iterates from 1 ton
(8 in this case) to generate and print the terms.
Output:
Number Series:
2 15 41 80 132 197 275 366
Key Points:
- Analyzing the pattern and deriving the formula is crucial for generating such non-standard series.
- The C code effectively implements the formula to produce the desired output.
- You can modify the value of
n
to print a different number of terms. - Consider adding comments within the code to explain the formula and logic for better readability.