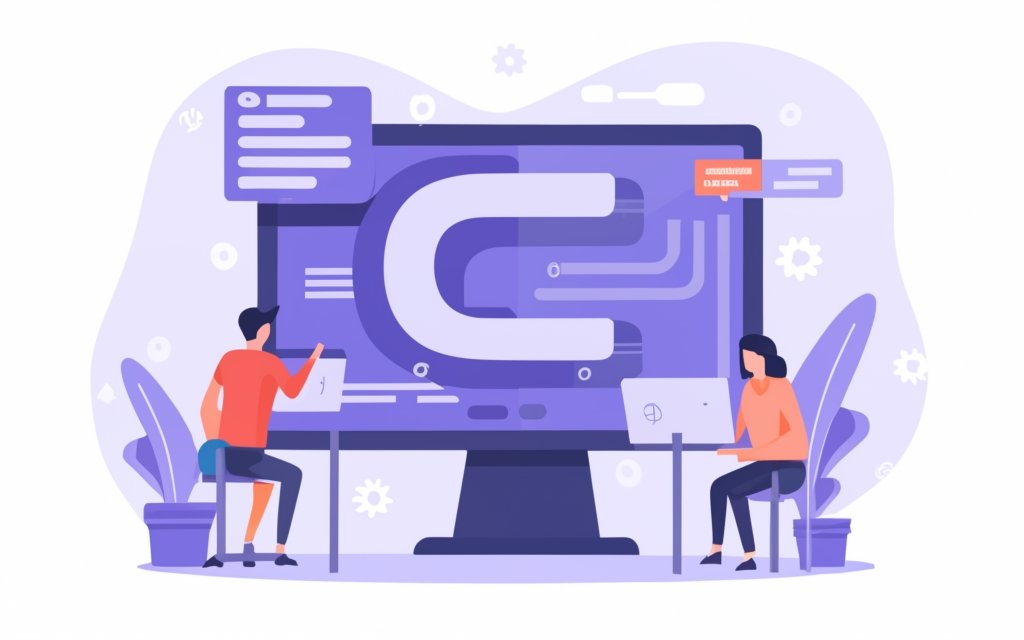
C (programming language)
C program to print 1 to n natural numbers
Understanding the Problem
- Goal: Write a C program that takes a positive integer
n
from the user and prints all natural numbers from 1 ton
. - Key Concepts:
- Variables: Containers for storing data (
n
). - Loops: Used to repeat code blocks (
for
loop in this case). - Input/Output (I/O): Communicating with the user (
scanf
,printf
).
- Variables: Containers for storing data (
Step-by-Step Guide
1. Header Inclusion
C
#include <stdio.h>
This line includes the standard input/output library, providing functions for printing to the console (e.g., printf
) and reading user input (e.g., scanf
).
2. Variable Declaration
C
int n;
This declares an integer variable named n
to store the user-provided positive integer.
3. Get User Input
C
printf("Enter a positive integer: ");
// Loop for input validation
do {
// Scan for integer input
if (scanf("%d", &n) != 1) {
printf("Invalid input. Please enter a positive integer: ");
// Clear input buffer to avoid infinite loop
scanf("%*c");
}
} while (n <= 0);
printf
: Prompts the user to enter a positive integer.do-while
loop: Ensures valid input.scanf("%d", &n)
: Reads the user’s input and stores it in then
variable.if (scanf("%d", &n) != 1)
: Checks if the scan was successful and if only one integer was entered.printf("Invalid input...")
: Informs the user of invalid input and loops again.scanf("%*c")
: Clears the input buffer from any leftover characters to avoid misreading in the next iteration.
4. Printing Numbers
C
// For loop to iterate from 1 to n
for (int i = 1; i <= n; i++) {
printf("%d\n", i);
}
for
loop:- Initializes
i
to 1. - Checks if
i
is less than or equal ton
. - Increments
i
by 1 each iteration.
- Initializes
printf("%d\n", i)
: Prints the current value ofi
(the loop counter) followed by a newline.
5. Complete Code
C
#include <stdio.h>
int main() {
int n;
printf("Enter a positive integer: ");
do {
if (scanf("%d", &n) != 1) {
printf("Invalid input. Please enter a positive integer: ");
scanf("%*c");
}
} while (n <= 0);
for (int i = 1; i <= n; i++) {
printf("%d\n", i);
}
return 0;
}
6. Executing the Program
- Save the code as a
.c
file (e.g.,print_naturals.c
). - Open a terminal or command prompt.
- Navigate to the directory where you saved the file.
- Compile the code using a C compiler (e.g.,
gcc print_naturals.c -o print_naturals
). - Run the compiled executable (e.g.,
./print_naturals
).
7. Example Output
Enter a positive integer: 5 1 2 3 4 5
This program is now complete and should function as intended.