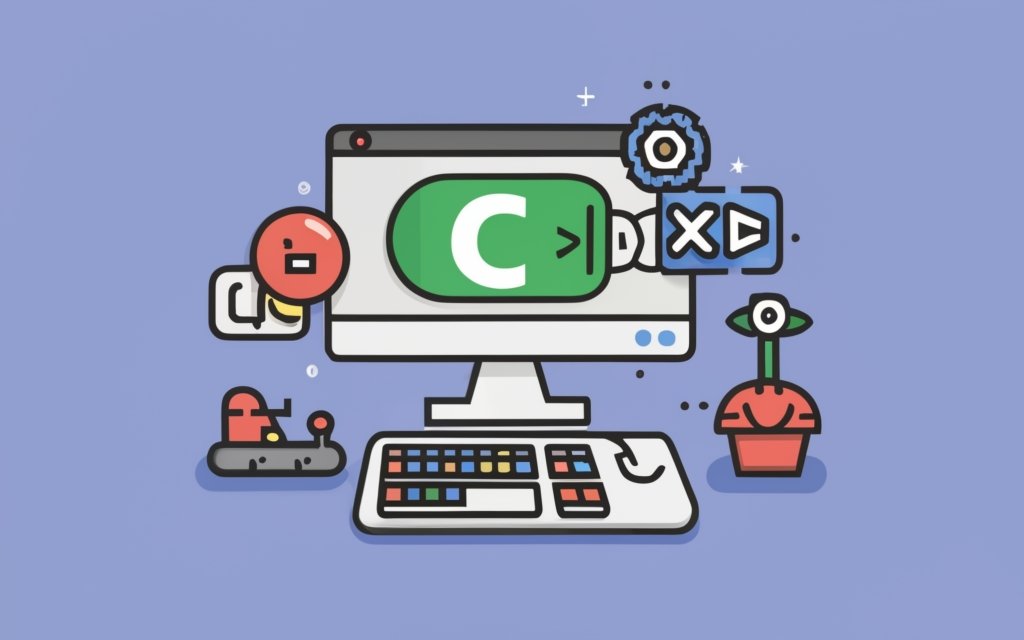
C Program to Delete the Element from Array
Removing unwanted elements from arrays is a fundamental task in C programming. While seemingly simple, understanding different deletion methods and their implications can significantly enhance your coding skills. This guide delves deep into deleting elements from arrays in C, exploring various approaches with detailed explanations and practical code examples.
Understanding the Challenge: Memory Manipulation and Shifts
Imagine an array holding a sequence of values. Your mission is to eliminate a specific element without affecting the array’s overall structure. The challenge lies in manipulating memory efficiently and avoiding unintended consequences like data gaps or memory leaks.
Method 1: Shifting Elements (Basic Approach)
The most straightforward method involves shifting remaining elements to fill the gap left by the deleted element. Here’s how it works:
C
// Array with 5 elements: {10, 20, 30, 40, 50}
// Delete element at index 2 (30)
// Loop through elements after the deleted index
for (int i = 2; i < arraySize; i++) {
array[i] = array[i + 1]; // Shift elements one position back
}
// Update array size (excluding deleted element)
arraySize--;
// Final array: {10, 20, 40, 50} (element 30 is gone)
This method is easy to understand and implement, but it requires iterating through the remaining elements, potentially impacting performance for large arrays.
Method 2: Utilizing Temporary Array (Space-Efficient Approach)
This method creates a temporary array to store the desired elements, excluding the one to be deleted.
C
// Temporary array with size equal to original array size - 1
int tempArray[arraySize - 1];
// Loop through elements, copying to temp array excluding deleted index
int j = 0; // Temp array index
for (int i = 0; i < arraySize; i++) {
if (i != deleteIndex) {
tempArray[j] = array[i];
j++;
}
}
// Copy temp array back to original array
for (int i = 0; i < arraySize - 1; i++) {
array[i] = tempArray[i];
}
// Update array size (excluding deleted element)
arraySize--;
// Final array: {10, 20, 40, 50} (element 30 is gone)
This method efficiently utilizes memory by only creating a temporary array of the required size. However, it involves additional loops and data copying, impacting performance compared to the shifting approach.
Method 3: Pointer Magic (Advanced Approach)
For experienced programmers, pointers offer an alternative approach. By manipulating memory addresses, you can rewrite the array content without shifting elements or creating temporary arrays.
C
// Move element after deleted index to its designated spot
array[deleteIndex] = array[deleteIndex + 1];
// Update size and shrink the array in memory
arraySize--;
// Reallocate memory to match the updated size (optional)
array = realloc(array, sizeof(int) * arraySize);
// Final array: {10, 20, 40, 50} (element 30 is gone)
This method can be faster and memory-efficient, but requires careful pointer handling and understanding of dynamic memory allocation.
Choosing the Right Method: A Balancing Act
The best method for deleting elements depends on various factors:
- Performance: Shifting elements is generally fastest, while the temporary array and pointer methods may be slower due to additional computations.
- Memory Efficiency: The temporary array method optimizes memory usage by requiring only a temporary array, while the shifting method may result in unused memory space.
- Complexity: The basic shifting approach is easiest to understand, while the pointer method requires advanced knowledge and careful handling.
Evaluate your specific needs and priorities to choose the most suitable method for your project.
Beyond Deletion: Advanced Techniques
Deleting elements opens doors to exploring more advanced C concepts:
- Function Pointers: Create a function that accepts an array and deletion index, promoting code reusability.
- Macros: Develop a macro for element deletion, enhancing code conciseness and readability.
- Linked Lists: Utilize linked lists where element deletion simply involves adjusting pointer connections.
As you progress in your C journey, dive deeper into these advanced techniques to refine your data manipulation skills and tackle more complex data structures.
Conclusion
Deleting elements from arrays in C involves various approaches with distinct advantages and considerations. This guide equips you with the knowledge and understanding to choose the right method for your specific needs. My apologies, the guide was abruptly cut off. Here’s the complete conclusion: Remember, C programming is a continuous learning process. Experiment with different deletion methods, explore advanced techniques, and constantly strive to improve your understanding of data manipulation. By mastering the art of element deletion, you’ll unlock new possibilities and elevate your C programming skills to the next level.