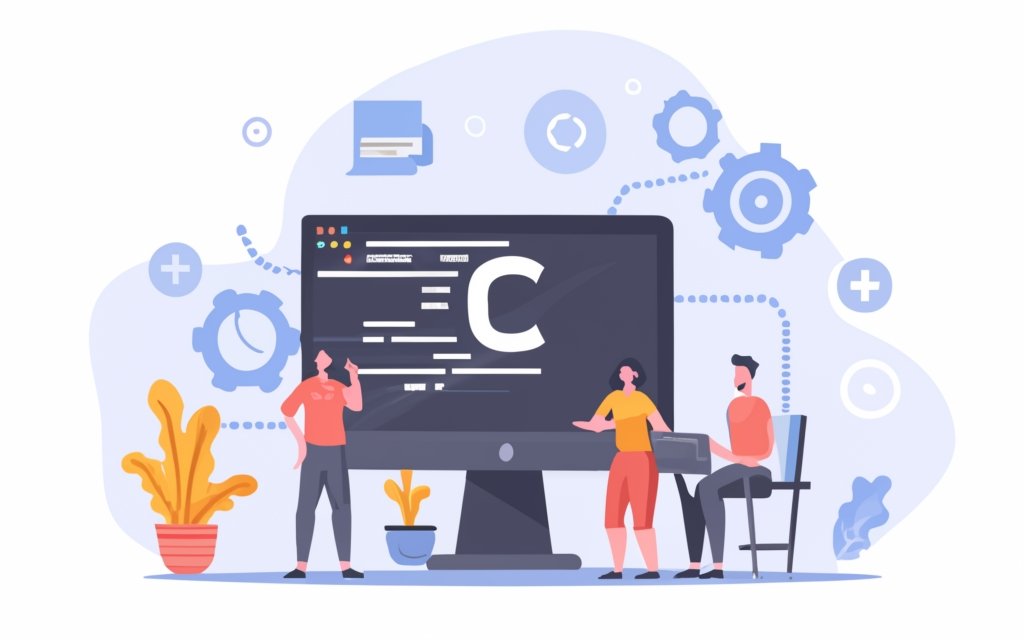
C Pattern Programming Exercises and Examples
C Pattern Programming Exercises and Examples: Unveiling the Beauty of Code
Pattern programming in C transcends mere data manipulation; it transforms lines of code into captivating visual displays. This guide dives into a collection of exercises and examples, unveiling the beauty and possibilities of crafting patterns with the versatile C language.
Diving into the Basics: Building Blocks of Pattern Magic
- Loops: Our trusty companions, loops control the repetition of code, guiding the construction of rows, columns, and diagonals for various patterns.
- Conditional Statements: These act as decision-makers, determining when to print characters like “*”, “#”, or spaces, shaping the desired pattern with precision.
- Variables: We utilize variables to store loop counters, pattern sizes, and other values, influencing the dynamic and varied nature of our creations.
1. Staircase Pattern: Climbing the Ladder of Code
The staircase pattern, a simple yet elegant example, showcases the power of nested loops and conditional statements.
C
for (int i = 1; i <= size; i++) { // Outer loop controls rows
for (int j = 1; j <= i; j++) { // Inner loop controls columns
printf("*"); // Print star for each column in a row
}
printf("\n"); // Move to new line after each row
}
This code demonstrates:
- Nested loops iterating based on the desired size.
- Inner loop printing stars for each column within a row.
- Outer loop adding line breaks to complete each row.
2. Diamond Pattern: Sparkling Gem of Symmetry
The diamond pattern, with its shimmering star outline and empty center, demands a nuanced approach to conditional statements.
C
for (int i = 1; i <= size; i++) { // Outer loop for upper half
for (int j = 1; j <= 2 * size - 1; j++) {
if (i == 1 || i == size || (j <= size - i + 1) && (j >= size + i - 1)) {
printf("*"); // Print star based on specific conditions
} else {
printf(" "); // Print space for hollow center and edges
}
}
printf("\n");
}
for (int i = size - 1; i >= 1; i--) { // Outer loop for lower half (similar logic as above)
// ...
}
This code showcases:
- Nested loops iterating for both halves of the diamond.
- Conditional statements controlling star placement based on row number and column position, ensuring the hollow center and diagonal borders.
- Mirrored logic for constructing the lower half of the diamond.
3. Pyramid Patterns: Ascending and Descending Triangles
Pyramids, representing ascent and descent, can be constructed with simple loop variations.
C
// Ascending Pyramid
for (int i = 1; i <= size; i++) {
for (int j = 1; j <= i; j++) {
printf("*");
}
printf("\n");
}
// Descending Pyramid (modified loop condition)
for (int i = size; i >= 1; i--) {
// ...
}
This code demonstrates:
- Ascending pyramid with nested loops increasing columns each row.
- Descending pyramid using a reversed outer loop for building downwards.
Beyond the Basics: Expanding Your Pattern Palette
C offers a vast canvas for pattern exploration. Here are some ideas to ignite your creativity:
- Diagonal Patterns: Experiment with loop conditions and variable adjustments to create diagonal lines of stars or other characters.
- Number Patterns: Incorporate numbers or letters within your patterns for visual interest and data representation.
- Animated Patterns: Utilize loop modifications and delay functions to create pulsating or moving patterns.
- User-Defined Patterns: Design your own patterns, drawing inspiration from nature, abstract art, or personal preferences.
Remember, practice makes perfect! Hone your skills by tackling these exercises and experimenting with different ideas. Soon, you’ll be weaving intricate patterns and dazzling creations with your C code, showcasing the artistic potential within every line.
Resources for Further Exploration:
- “C Programming Exercises and Solutions” by Brian Kernighan and Dennis Ritchie
- “Learn C the Hard Way” by Zed A. Shaw
- Online forums and communities dedicated to C programming
The journey of mastering pattern programming in C is just beginning. Embrace the challenge, unleash your creativity, and let your code blossom into vibrant visual artistry!